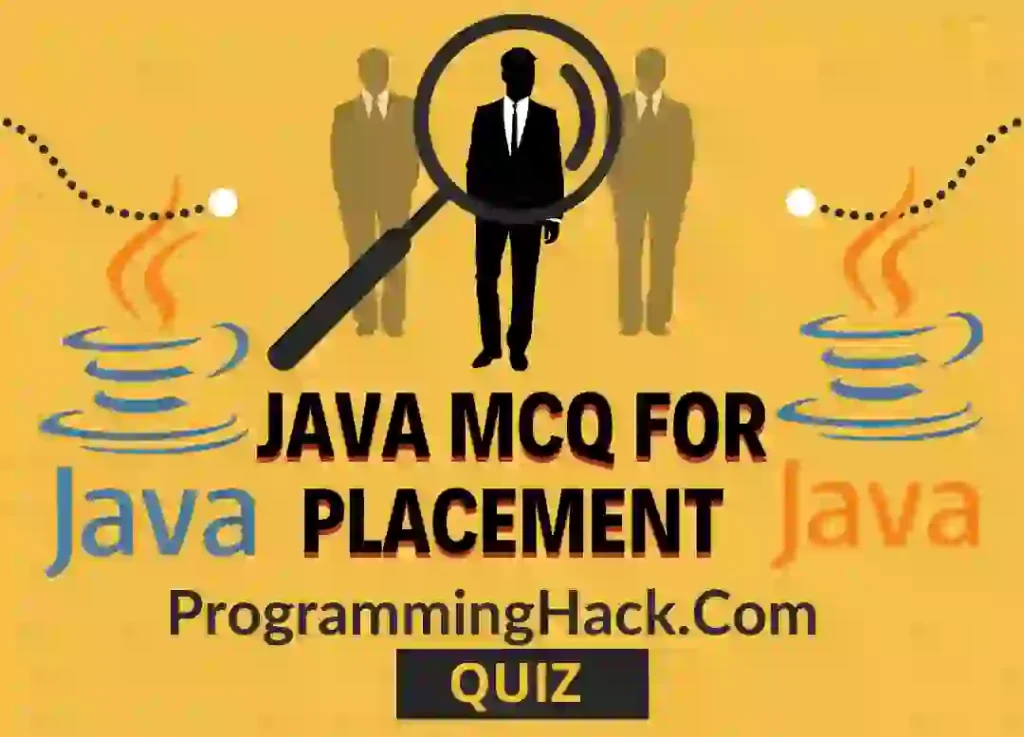
Java MCQ for placement questions with answers:
Crack Your Coding Interviews
Hey there! Ready to dive into the world of Java? Our java MCQ for placement series is here to make learning fun and easy, especially if you’re gearing up for placements. Java is like the Swiss Army knife of programming languages, super versatile and used everywhere in software development. Whether you’re just starting out or already a pro, our MCQs will help you brush up on your Java skills. Let’s get cracking and ace those placements!
Q.1 What is the result of the following code snippet?
int x = 10;
int y = 20;
int z = (x > y) ? x++ : y–;
System.out.println(z);
a) 10
b) 20
c) 21
d) 19
Answer: a) 10
Explanation: The conditional operator evaluates to the value of the expression on the true side if the condition is true, otherwise to the value of the expression on the false side. Here, since x > y is false, y– is evaluated and the value of y (20) is assigned to z.
Q.2 Which of the following statements about Java interfaces is false?
a) Interfaces can have constructors.
b) Interfaces can extend other interfaces.
c) Interfaces can have static methods.
d) Interfaces can have default methods.
Answer: a) Interfaces can have constructors.
Explanation: Interfaces cannot have constructors because they cannot be instantiated directly. They are implemented by classes, which provide their own constructors.
Q.3 What will be the output of the following code snippet?
int[] array = {1, 2, 3};
int sum = 0;
for (int i = 0; i <= array.length; i++) {
sum += array[i];
}
System.out.println(sum);
a) 6
b) 7
c) Compilation error
d) ArrayIndexOutOfBoundsException
Answer: d) ArrayIndexOutOfBoundsException
Explanation: The loop condition should be i < array.length instead of i <= array.length to prevent accessing an index beyond the bounds of the array.
Q.4 Which of the following statements about Java generics is true?
a) Generics allow runtime type checking.
b) Generics can be used with primitive data types.
c) Generics support covariant arrays.
d) Generics cannot be used with collections.
Answer: b) Generics can be used with primitive data types.
Explanation: Generics in Java can be used with reference types, including wrapper classes for primitive types, but not with primitive types themselves.
Q.5 What is the purpose of the ‘volatile’ keyword in Java?
a) It prevents a variable from being modified by multiple threads.
b) It ensures that a variable is visible to all threads.
c) It ensures that a variable’s value is never cached thread-locally.
d) It guarantees that a variable is initialized before it is accessed.
Answer: c) It ensures that a variable’s value is never cached thread-locally.
Explanation: The ‘volatile’ keyword in Java ensures that the value of a variable is always read from and written to main memory, rather than being cached thread-locally.
Q.6 What is the output of the following code snippet?
String str1 = “hello”;
String str2 = “hello”;
System.out.println(str1 == str2);
a) true
b) false
c) Compilation error
d) Runtime error
Answer: a) true
Explanation: String literals with the same value are interned, meaning they refer to the same object in memory. Therefore, str1 and str2 point to the same object.
Q.7 What does the ‘transient’ keyword do in Java?
a) It specifies that a variable cannot be modified.
b) It specifies that a variable is not to be serialized.
c) It specifies that a variable can only be accessed by methods within its own class.
d) It specifies that a variable can have a value of null.
Answer: b) It specifies that a variable is not to be serialized.
Explanation: The ‘transient’ keyword in Java is used to indicate that a variable should not be included in the serialization process.
Q.8 What is the purpose of the ‘strictfp’ keyword in Java?
a) It ensures that a class cannot be subclassed.
b) It restricts access to a method or variable.
c) It forces floating-point calculations to adhere to the IEEE 754 standard.
d) It enables assertions for a class.
Answer: c) It forces floating-point calculations to adhere to the IEEE 754 standard.
Explanation: The ‘strictfp’ keyword in Java ensures that floating-point calculations are performed in accordance with the IEEE 754 standard, providing platform-independent results.
Q.9 Which of the following is not a valid declaration of a Java method?
a) void method();
b) int method(int x);
c) static void method();
d) final int method();
Answer: d) final int method();
Explanation: Methods cannot be declared as both ‘final’ and ‘void’ or ‘final’ and have a return type.
Q.10 What is the output of the following code snippet?
int x = 5;
System.out.println(x++ * ++x);
a) 25
b) 30
c) 35
d) 40
Answer: c) 35
Explanation: The expression evaluates to 5 * 7 because x++ is post-incremented, so it uses the current value of x (5), and ++x is pre-incremented, so it uses the incremented value of x (6).
Core Java MCQ for placement Collection of MCQs for Basic Placement Preparation
In today’s competitive job market, having a solid grasp of Java programming is crucial, especially for those aiming to land entry-level positions. To stand out in placement exams and interviews, it’s essential to have a strong foundation in Core Java concepts. This blog is designed to be a helpful resource for budding Java developers, offering a curated set of java MCQ for placement focusing on key Core Java topics. Whether you’re a recent graduate gearing up for your first job interview or a seasoned professional looking to refresh your basics, these MCQs will be a valuable tool to reinforce your understanding and feel more confident when facing placement assessments.
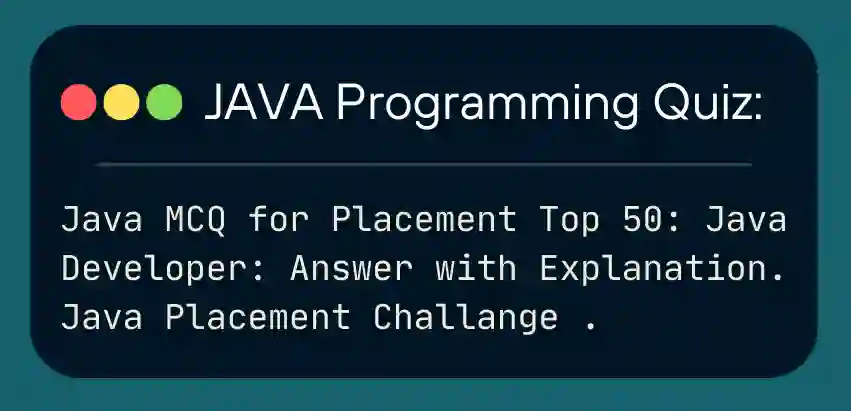
Q.1 Which of the following statements about Java constructors is true?
a) Constructors can be inherited.
b) Constructors can have a return type.
c) Constructors can be declared as final.
d) Constructors can be overloaded.
Answer: d) Constructors can be overloaded.
Explanation: Constructors in Java can be overloaded, meaning a class can have multiple constructors with different parameter lists.
Q.2 What is the output of the following code snippet?
int x = 10;
System.out.println(x++ + ++x);
a) 21
b) 22
c) 23
d) 24
Answer: c) 23
Explanation: The expression evaluates to 10 + 12 because x++ is post-incremented, so it uses the current value of x (10
Q.3 Which of the following is not a valid access modifier in Java?
a) private
b) protected
c) internal
d) public
Answer: c) internal
Explanation: ‘internal’ is not a valid access modifier in Java. The correct modifiers are private, protected, and public.
Q.4 What is the output of the following code snippet?
int[] numbers = {1, 2, 3, 4, 5};
int sum = 0;
for (int i = 0; i < numbers.length; i++) {
if (i % 2 == 0) {
continue;
}
sum += numbers[i];
}
System.out.println(sum);
a) 3
b) 6
c) 9
d) 12
Answer: b) 6
Explanation: The loop iterates over odd indices (i % 2 == 0) and adds their values to the sum.
Q.5 What is the purpose of the ‘finally’ block in Java exception handling?
a) It ensures that certain code is always executed, regardless of whether an exception is thrown or caught.
b) It specifies code that is executed when an exception occurs.
c) It indicates a block of code that should be skipped if an exception occurs.
d) It catches exceptions thrown by try blocks.
Answer: a) It ensures that certain code is always executed, regardless of whether an exception is thrown or caught.
Explanation: The ‘finally’ block in Java is used to ensure that certain code is always executed, regardless of whether an exception is thrown or caught.
Q.6 What is the correct syntax for a conditional (ternary) operator in Java?
a) if (condition) ? value1 : value2;
b) condition ? value1 : value2;
c) if (condition) then value1 else value2;
d) condition then value1 else value2;
Answer: b) condition ? value1 : value2;
Explanation: The ternary operator in Java has the syntax: condition ? value1 : value2.
Q.7 Which of the following statements about Java collections is true?
a) Arrays are part of the Java Collections Framework.
b) HashSet allows duplicate elements.
c) LinkedList is a synchronized collection.
d) TreeMap is not a sorted collection.
Answer: a) Arrays are part of the Java Collections Framework.
Explanation: Arrays are part of the Java Collections Framework, but they are not part of the java.util package.
Q.8 What is the purpose of the ‘static’ keyword in Java?
a) It makes a variable constant.
b) It enables methods and variables to belong to the class rather than an instance.
c) It ensures that a method cannot be overridden.
d) It allows access to the superclass methods and variables.
Answer: b) It enables methods and variables to belong to the class rather than an instance.
Explanation: The ‘static’ keyword in Java is used to declare methods and variables that belong to the class itself, rather than to instances of the class.
Q.9 Which of the following is not a valid Java identifier?
a) _variableName
b) 2variable
c) $variable
d) variableName
Answer: b) 2variable
Explanation: Java identifiers cannot start with a digit.
Q.10 What is the output of the following code snippet?
String str = “Hello”;
str.concat(” World”);
System.out.println(str);
a) Hello
b) World
c) Hello World
d) Null
Answer: a) Hello
Explanation: Strings in Java are immutable, so the concat() method returns a new string without modifying the original one.
Java MCQs for Placement Success: Mastering Java Programming Concepts

Prepare for your placement interviews with our comprehensive collection of Java Multiple Choice Questions (MCQs). From basic concepts to advanced topics, test your knowledge and boost your confidence in Java programming for successful placements.
What is an exception in Java?
a) A syntax error detected by the compiler
b) An event occurring during the execution of a program that disrupts the normal flow of instructions
c) A warning issued by the Java Virtual Machine (JVM)
d) An error caused by hardware malfunction
Answer: b) An event occurring during the execution of a program that disrupts the normal flow of instructions
Explanation: An exception in Java is an event that occurs during the execution of a program and disrupts the normal flow of instructions.
Which keyword is used to handle exceptions in Java?
a) try
b) catch
c) throw
d) all of the above
Answer: d) all of the above
Explanation: In Java, exceptions are handled using the try-catch blocks, and exceptions can also be thrown using the ‘throw’ keyword.
What is the purpose of the ‘finally’ block in exception handling?
a) To catch exceptions
b) To define code that will always be executed, regardless of whether an exception occurs or not
c) To throw exceptions
d) To handle checked exceptions
Answer: b) To define code that will always be executed, regardless of whether an exception occurs or not
Explanation: The ‘finally’ block in Java is used to define code that will always be executed, regardless of whether an exception occurs or not.
Which of the following exceptions is a checked exception in Java?
a) ArithmeticException
b) NullPointerException
c) IOException
d) ArrayIndexOutOfBoundsException
Answer: c) IOException
Explanation: IOException is a checked exception in Java, which means that it must be either caught or declared to be thrown by the method in which it may occur.
What is the output of the following code snippet?
try {
int num = Integer.parseInt(“ABC”);
System.out.println(num);
} catch (NumberFormatException e) {
System.out.println(“NumberFormatException occurred”);
}
a) ABC
b) NumberFormatException occurred
c) 0
d) Compilation error
Answer: b) NumberFormatException occurred
Explanation: The parseInt() method throws a NumberFormatException if the string cannot be parsed as an integer, so the catch block will be executed, printing “NumberFormatException occurred”.
Which of the following is NOT a standard exception class in Java?
a) RuntimeException
b) Exception
c) Throwable
d) Error
Answer: a) RuntimeException
Explanation: RuntimeException is a subclass of Exception and is used to represent exceptions that occur during the normal operation of the Java Virtual Machine (JVM).
What happens if an exception is thrown from within a ‘finally’ block?
a) The exception is caught by the nearest enclosing try-catch block.
b) The program terminates.
c) The exception is ignored.
d) The finally block is not executed.
Answer: a) The exception is caught by the nearest enclosing try-catch block.
Explanation: If an exception is thrown from within a ‘finally’ block, it is caught by the nearest enclosing try-catch block that can handle it.
Which keyword is used to explicitly throw an exception in Java?
a) try
b) catch
c) throw
d) throws
Answer: c) throw
Explanation: The ‘throw’ keyword is used to explicitly throw an exception in Java.
What is the difference between checked and unchecked exceptions in Java?
a) Checked exceptions are checked at compile time, while unchecked exceptions are checked at runtime.
b) Checked exceptions are checked at runtime, while unchecked exceptions are checked at compile time.
c) Checked exceptions are subclasses of RuntimeException, while unchecked exceptions are not.
d) Checked exceptions can be caught using a try-catch block, while unchecked exceptions cannot.
Answer: a) Checked exceptions are checked at compile time, while unchecked exceptions are checked at runtime.
Explanation: Checked exceptions are checked at compile time, meaning that the compiler ensures that they are either caught or declared to be thrown. Unchecked exceptions, on the other hand, are not checked at compile time and are typically subclasses of RuntimeException.
What is the superclass of all exception classes in Java?
a) Error
b) Throwable
c) Exception
d) RuntimeException
Answer: b) Throwable
Explanation: Throwable is the superclass of all exception classes in Java. Both exceptions and errors are subclasses of Throwable.
10 Must-Know MCQs for Technical Interviews (with Solutions) Java MCQ for Placement
Prepare for your placement exams confidently with our carefully selected Java MCQ for Placement. Our comprehensive collection is designed to help you master essential Java concepts, ensuring you’re well-equipped for your upcoming interviews. Test your knowledge, identify any areas that need improvement, and boost your confidence for placement assessments with ease.
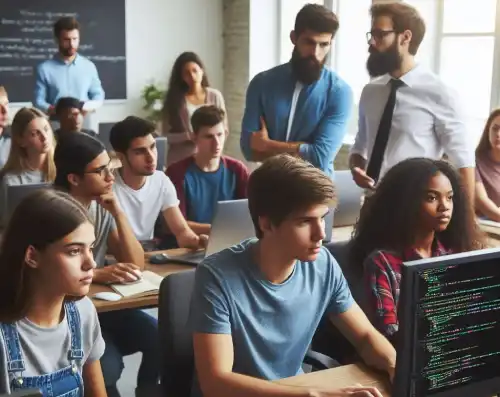
What is the time complexity of accessing an element in an array by its index?
a) O(1)
b) O(log n)
c) O(n)
d) O(n^2)
Answer: a) O(1)
Explanation: Accessing an element in an array by its index has a time complexity of O(1) because arrays provide constant-time random access to elements.
Which data structure follows the Last In First Out (LIFO) principle?
a) Queue
b) Stack
c) Linked List
d) Heap
Answer: b) Stack
Explanation: Stacks follow the Last In First Out (LIFO) principle, where the last element added is the first one to be removed.
In a binary search tree (BST), which traversal method visits the root node between the left and right subtrees?
a) Preorder
b) Inorder
c) Postorder
d) Level order
Answer: b) Inorder
Explanation: In an inorder traversal of a binary search tree (BST), the nodes are visited in the order: left, root, right.
Which sorting algorithm has the best time complexity in the worst-case scenario?
a) Bubble Sort
b) Insertion Sort
c) Merge Sort
d) Selection Sort
Answer: c) Merge Sort
Explanation: Merge Sort has a time complexity of O(n log n) in the worst-case scenario, which is better than the quadratic time complexities of Bubble Sort, Insertion Sort, and Selection Sort.
What is the time complexity of inserting an element at the end of a singly linked list?
a) O(1)
b) O(log n)
c) O(n)
d) O(n^2)
Answer: a) O(1)
Explanation: Inserting an element at the end of a singly linked list has a time complexity of O(1) because it involves updating the next pointer of the last node to point to the new node.
Which data structure allows access to its elements in a non-linear order using keys?
a) Array
b) Stack
c) Queue
d) Dictionary
Answer: d) Dictionary
Explanation: A dictionary (or map) allows access to its elements in a non-linear order using keys for efficient retrieval.
Which data structure is used to implement priority queues?
a) Stack
b) Queue
c) Heap
d) Linked List
Answer: c) Heap
Explanation: Heaps are often used to implement priority queues because they allow efficient insertion and removal of the highest (or lowest) priority element.
Which search algorithm is typically used for searching in a sorted array?
a) Linear Search
b) Binary Search
c) Depth-First Search
d) Breadth-First Search
Answer: b) Binary Search
Explanation: Binary Search is typically used for searching in a sorted array because it has a time complexity of O(log n), which is much better than the linear time complexity of Linear Search.
What is the primary disadvantage of using a linked list over an array?
a) Linked lists have slower access time.
b) Linked lists require contiguous memory allocation.
c) Linked lists have limited flexibility in inserting and deleting elements.
d) Linked lists use more memory.
Answer: a) Linked lists have slower access time.
Explanation: Linked lists have slower access time compared to arrays because accessing an element in a linked list requires traversing the list from the beginning.
In a heap data structure, what is the relationship between a parent node and its children?
a) Each child node is smaller than its parent node.
b) Each child node is greater than its parent node.
c) Each child node is equal to its parent node.
d) There is no specific relationship between a parent node and its children in a heap.
Answer: a) Each child node is smaller than its parent node.
Explanation: In a min-heap, each child node is smaller than its parent node, maintaining the heap property where the root node is the minimum element. Similarly, in a max-heap, each child node is greater than its parent node.
For learning Java Programming through multiple-choice questions, consider exploring resources
For additional resources to learn Java Programming through java mcq questions & mcq on inheritance in java, you can explore various online platforms and websites dedicated to programming education and assessment. Here are some suggestions:
- Core JAVA MCQ Questions Test with Answer-Top 50 – Programming Hack
- Core Java MCQ – Top 100 Questions and Answers (javaguides.net)
- 50 Java MCQ Questions Interview Questions: Cracking Java Interviews – Programming Hack
- JAVA MCQ Questions Online Test, Exam, Quiz, and Practice Top 100+ – Programming Hack
- Master the Java Interview: Top 100 Java MCQ Questions and Answers – Programming Hack
- MCQ on Inheritance in java: Top 30 – Programming Hack
By exploring these resources, you can find a variety of Java MCQ Questions Answer to practice and enhance your Java programming skills at your own pace.
1 thought on “Java MCQ for Placement Top 100: Strong Your Coding Interviews:”